top of page
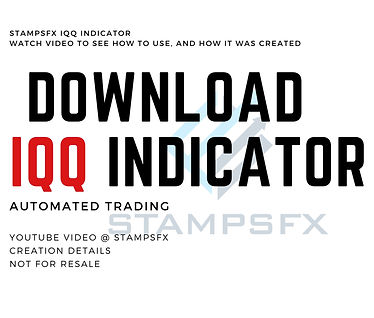
Thank you for visiting. Before you copy the code below be sure to subscribe to the YouTube channel 'StampsFX' and like the videos.
Copy the below code and paste into Meta Editor as instructed in the video.
​
//+------------------------------------------------------------------+
//| Indicator: IQQ.mq4 |
//| Created with EABuilder.com |
//| https://www.eabuilder.com |
//+------------------------------------------------------------------+
#property copyright "Created with EABuilder.com"
#property link "https://www.eabuilder.com"
#property version "1.00"
#property description ""
#include <stdlib.mqh>
#include <stderror.mqh>
//--- indicator settings
#property indicator_chart_window
#property indicator_buffers 4
#property indicator_type1 DRAW_ARROW
#property indicator_width1 1
#property indicator_color1 0x1100FF
#property indicator_label1 "SELL"
#property indicator_type2 DRAW_ARROW
#property indicator_width2 1
#property indicator_color2 0xFF3700
#property indicator_label2 "BUY"
#property indicator_type3 DRAW_ARROW
#property indicator_width3 3
#property indicator_color3 0x0004FF
#property indicator_label3 "SELL"
#property indicator_type4 DRAW_ARROW
#property indicator_width4 3
#property indicator_color4 0xFF0004
#property indicator_label4 "BUY"
//--- indicator buffers
double Buffer1[];
double Buffer2[];
double Buffer3[];
double Buffer4[];
double myPoint; //initialized in OnInit
void myAlert(string type, string message)
{
if(type == "print")
Print(message);
else if(type == "error")
{
Print(type+" | IQQ @ "+Symbol()+","+IntegerToString(Period())+" | "+message);
}
else if(type == "order")
{
}
else if(type == "modify")
{
}
}
void DrawLine(string objname, double price, int count, int start_index) //creates or modifies existing object if necessary
{
if((price < 0) && ObjectFind(objname) >= 0)
{
ObjectDelete(objname);
}
else if(ObjectFind(objname) >= 0 && ObjectType(objname) == OBJ_TREND)
{
ObjectSet(objname, OBJPROP_TIME1, Time[start_index]);
ObjectSet(objname, OBJPROP_PRICE1, price);
ObjectSet(objname, OBJPROP_TIME2, Time[start_index+count-1]);
ObjectSet(objname, OBJPROP_PRICE2, price);
}
else
{
ObjectCreate(objname, OBJ_TREND, 0, Time[start_index], price, Time[start_index+count-1], price);
ObjectSet(objname, OBJPROP_RAY, false);
ObjectSet(objname, OBJPROP_COLOR, C'0x00,0x00,0xFF');
ObjectSet(objname, OBJPROP_STYLE, STYLE_SOLID);
ObjectSet(objname, OBJPROP_WIDTH, 2);
}
}
double Support(int time_interval, bool fixed_tod, int hh, int mm, bool draw, int shift)
{
int start_index = shift;
int count = time_interval / 60 / Period();
if(fixed_tod)
{
datetime start_time;
if(shift == 0)
start_time = TimeCurrent();
else
start_time = Time[shift-1];
datetime dt = StringToTime(StringConcatenate(TimeToString(start_time, TIME_DATE)," ",hh,":",mm)); //closest time hh:mm
if (dt > start_time)
dt -= 86400; //go 24 hours back
int dt_index = iBarShift(NULL, 0, dt, true);
datetime dt2 = dt;
while(dt_index < 0 && dt > Time[Bars-1-count]) //bar not found => look a few days back
{
dt -= 86400; //go 24 hours back
dt_index = iBarShift(NULL, 0, dt, true);
}
if (dt_index < 0) //still not found => find nearest bar
dt_index = iBarShift(NULL, 0, dt2, false);
start_index = dt_index + 1; //bar after S/R opens at dt
}
double ret = Low[iLowest(NULL, 0, MODE_LOW, count, start_index)];
if (draw) DrawLine("Support", ret, count, start_index);
return(ret);
}
double Resistance(int time_interval, bool fixed_tod, int hh, int mm, bool draw, int shift)
{
int start_index = shift;
int count = time_interval / 60 / Period();
if(fixed_tod)
{
datetime start_time;
if(shift == 0)
start_time = TimeCurrent();
else
start_time = Time[shift-1];
datetime dt = StringToTime(StringConcatenate(TimeToString(start_time, TIME_DATE)," ",hh,":",mm)); //closest time hh:mm
if (dt > start_time)
dt -= 86400; //go 24 hours back
int dt_index = iBarShift(NULL, 0, dt, true);
datetime dt2 = dt;
while(dt_index < 0 && dt > Time[Bars-1-count]) //bar not found => look a few days back
{
dt -= 86400; //go 24 hours back
dt_index = iBarShift(NULL, 0, dt, true);
}
if (dt_index < 0) //still not found => find nearest bar
dt_index = iBarShift(NULL, 0, dt2, false);
start_index = dt_index + 1; //bar after S/R opens at dt
}
double ret = High[iHighest(NULL, 0, MODE_HIGH, count, start_index)];
if (draw) DrawLine("Resistance", ret, count, start_index);
return(ret);
}
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
IndicatorBuffers(4);
SetIndexBuffer(0, Buffer1);
SetIndexEmptyValue(0, EMPTY_VALUE);
SetIndexArrow(0, 242);
SetIndexBuffer(1, Buffer2);
SetIndexEmptyValue(1, EMPTY_VALUE);
SetIndexArrow(1, 241);
SetIndexBuffer(2, Buffer3);
SetIndexEmptyValue(2, EMPTY_VALUE);
SetIndexArrow(2, 252);
SetIndexBuffer(3, Buffer4);
SetIndexEmptyValue(3, EMPTY_VALUE);
SetIndexArrow(3, 252);
//initialize myPoint
myPoint = Point();
if(Digits() == 5 || Digits() == 3)
{
myPoint *= 10;
}
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int OnCalculate(const int rates_total,
const int prev_calculated,
const datetime& time[],
const double& open[],
const double& high[],
const double& low[],
const double& close[],
const long& tick_volume[],
const long& volume[],
const int& spread[])
{
int limit = rates_total - prev_calculated;
//--- counting from 0 to rates_total
ArraySetAsSeries(Buffer1, true);
ArraySetAsSeries(Buffer2, true);
ArraySetAsSeries(Buffer3, true);
ArraySetAsSeries(Buffer4, true);
//--- initial zero
if(prev_calculated < 1)
{
ArrayInitialize(Buffer1, EMPTY_VALUE);
ArrayInitialize(Buffer2, EMPTY_VALUE);
ArrayInitialize(Buffer3, EMPTY_VALUE);
ArrayInitialize(Buffer4, EMPTY_VALUE);
}
else
limit++;
//--- main loop
for(int i = limit-1; i >= 0; i--)
{
if (i >= MathMin(5000-1, rates_total-1-50)) continue; //omit some old rates to prevent "Array out of range" or slow calculation
//Indicator Buffer 1
if(iStochastic(NULL, PERIOD_CURRENT, 5, 3, 3, MODE_SMA, 0, MODE_MAIN, i) < 80
&& iStochastic(NULL, PERIOD_CURRENT, 5, 3, 3, MODE_SMA, 0, MODE_MAIN, i+1) > 80 //Stochastic Oscillator crosses below fixed value
)
{
Buffer1[i] = Resistance(12 * PeriodSeconds(), false, 00, 00, false, i); //Set indicator value at Resistance
}
else
{
Buffer1[i] = EMPTY_VALUE;
}
//Indicator Buffer 2
if(iStochastic(NULL, PERIOD_CURRENT, 5, 3, 3, MODE_SMA, 0, MODE_MAIN, i) > 20
&& iStochastic(NULL, PERIOD_CURRENT, 5, 3, 3, MODE_SMA, 0, MODE_MAIN, i+1) < 20 //Stochastic Oscillator crosses above fixed value
)
{
Buffer2[i] = Support(12 * PeriodSeconds(), false, 00, 00, false, i); //Set indicator value at Support
}
else
{
Buffer2[i] = EMPTY_VALUE;
}
//Indicator Buffer 3
if(iMA(NULL, PERIOD_CURRENT, 10, 0, MODE_EMA, PRICE_CLOSE, i) < iMA(NULL, PERIOD_CURRENT, 40, 0, MODE_EMA, PRICE_CLOSE, i)
&& iMA(NULL, PERIOD_CURRENT, 10, 0, MODE_EMA, PRICE_CLOSE, i+1) > iMA(NULL, PERIOD_CURRENT, 40, 0, MODE_EMA, PRICE_CLOSE, i+1) //Moving Average crosses below Moving Average
)
{
Buffer3[i] = Resistance(12 * PeriodSeconds(), false, 00, 00, false, i); //Set indicator value at Resistance
}
else
{
Buffer3[i] = EMPTY_VALUE;
}
//Indicator Buffer 4
if(iMA(NULL, PERIOD_CURRENT, 10, 0, MODE_EMA, PRICE_CLOSE, i) > iMA(NULL, PERIOD_CURRENT, 40, 0, MODE_EMA, PRICE_CLOSE, i)
&& iMA(NULL, PERIOD_CURRENT, 10, 0, MODE_EMA, PRICE_CLOSE, i+1) < iMA(NULL, PERIOD_CURRENT, 40, 0, MODE_EMA, PRICE_CLOSE, i+1) //Moving Average crosses above Moving Average
)
{
Buffer4[i] = Support(12 * PeriodSeconds(), false, 00, 00, false, i); //Set indicator value at Support
}
else
{
Buffer4[i] = EMPTY_VALUE;
}
}
return(rates_total);
}
//+------------------------------------------------------------------+
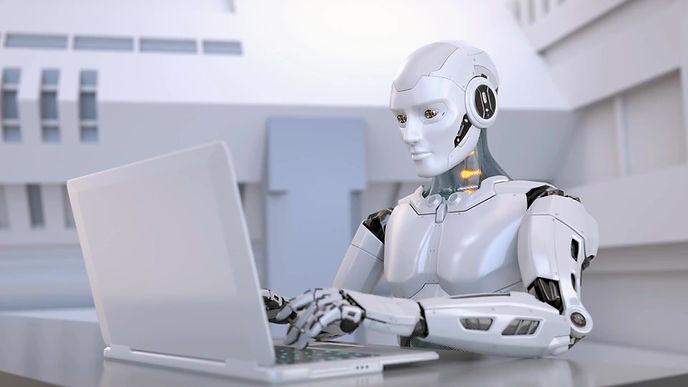
bottom of page